Essential Programming Challenges
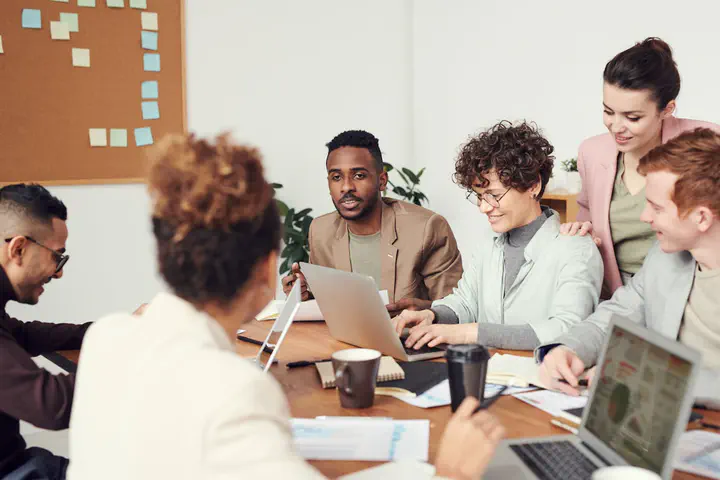
Explore a diverse range of programming challenges, including grade calculators, weather forecasts, and employee bonus systems. Perfect for honing your coding skills and applying concepts in practical scenarios.
More Programs for Practice
1️⃣ Employee Bonus Calculator
💼 Write a program that takes the employee’s years of service and job type as input and outputs the bonus amount based on the following conditions:
- 🏆 years of service above 10 and job type manager: ₹50000
- 🏅 years of service above 10 and job type non-manager: ₹20000
- ⏳ years of service below 10 and job type manager: ₹20000
- ⏳ years of service below 10 and job type non-manager: ₹10000
2️⃣ Weather Forecast
🌡️ Write a program that takes the temperature as input and outputs the weather forecast based on the following conditions:
- 🔥 Hot: above 85
- ☀️ Warm: 65 - 84
- 🌬️ Cool: 55 - 64
- ❄️ Cold: below 55
3️⃣ Discount Calculator
💰 Write a program that takes the price and discount percentage as input and outputs the discounted price based on the following conditions:
- 🔟 10% discount for prices above ₹100
- 5️⃣ 5% discount for prices between ₹50 - ₹99
- 🚫 No discount for prices below ₹50
4️⃣ Age Category
👶 Write a program that takes the age as input and outputs the corresponding age category based on the following conditions:
- 👶 Child: 0 - 12
- 👦 Teenager: 13 - 19
- 👨 Adult: 20 - 64
- 👴 Senior: 65 and above
5️⃣ Time of Day
🕒 Write a program that takes the time in hours as input and outputs the corresponding time of day based on the following conditions:
- 🌅 Morning: 6 - 11
- ☀️ Afternoon: 12 - 16
- 🌇 Evening: 17 - 20
- 🌙 Night: 21 - 5
6️⃣ Color Code
🎨 Write a program that takes a color code as input and outputs the corresponding color name based on the following conditions:
- 1️⃣ 1: Red
- 2️⃣ 2: Green
- 3️⃣ 3: Blue
- 4️⃣ 4: Yellow
7️⃣ Status Code
📊 Write a program that takes a status code as input and outputs the corresponding status message based on the following conditions:
- 2️⃣0️⃣0️⃣: Success
- 4️⃣0️⃣4️⃣: Not Found
- 5️⃣0️⃣0️⃣: Internal Server Error
8️⃣ Score Range
🏆 Write a program that takes a score as input and outputs the corresponding score range based on the following conditions:
- 90 - 100: Excellent
- 80 - 89: Good
- 70 - 79: Fair
- 60 - 69: Poor
- below 60: Fail
9️⃣ Temperature Scale
🌡️ Write a program that takes a temperature in Celsius as input and outputs the corresponding temperature in Fahrenheit based on the following conditions:
- above 0: Fahrenheit = Celsius * 9/5 + 32
- below 0: Fahrenheit = Celsius * 9/5 - 32
🔟 Student Eligibility
🎓 Write a program that takes the student’s GPA and test scores as input and outputs whether they are eligible for a scholarship based on the following conditions:
- GPA above 3.5 and test score above 80: Eligible
- GPA above 3.0 and test score above 70: Maybe
- Otherwise: Not Eligible
1️⃣1️⃣ Bank Account Type
🏦 Write a program that takes the account balance as input and outputs the corresponding account type based on the following conditions:
- above ₹10000: Premium
- between ₹1000 - ₹9999: Standard
- below ₹1000: Basic
1️⃣2️⃣ Shipping Cost
📦 Write a program that takes the package weight and distance as input and outputs the shipping cost based on the following conditions:
- weight above 10kg and distance above 1000km: ₹50
- weight above 10kg and distance below 1000km: ₹30
- weight below 10kg and distance above 1000km: ₹40
- weight below 10kg and distance below 1000km: ₹20
1️⃣3️⃣ Employee Bonus
💼 Write a program that takes the employee’s salary and years of service as input and outputs the corresponding bonus based on the following conditions:
- salary above ₹50000 and years of service above 5: 10% bonus
- salary above ₹30000 and years of service above 3: 5% bonus
- otherwise: no bonus
1️⃣4️⃣ Student Grade Level
📚 Write a program that takes the student’s age as input and outputs the corresponding grade level based on the following conditions:
- above 17: College
- between 14 - 16: High School
- between 11 - 13: Middle School
- between 6 - 10: Elementary School
- below 6: Preschool
1️⃣5️⃣ Tax Bracket
💵 Write a program that takes the taxpayer’s income as input and outputs the corresponding tax bracket based on the following conditions:
- above ₹100000: 20%
- between ₹50000 - ₹99999: 15%
- between ₹20000 - ₹49999: 10%
- below ₹20000: 5%
1️⃣6️⃣ Credit Card Approval
💳 Write a program that takes the applicant’s credit score and income as input and outputs whether they are approved for a credit card based on the following conditions:
- credit score above 700 and income above ₹50000: Approved
- credit score above 600 and income above ₹30000: Maybe
- otherwise: Denied
1️⃣7️⃣ Hotel Room Booking
🏨 Write a program that takes the room type and number of guests as input and outputs the total cost based on the following conditions:
- luxury room and more than 2 guests: ₹200 per night
- standard room and more than 2 guests: ₹150 per night
- luxury room and 1 - 2 guests: ₹180 per night
- standard room and 1 - 2 guests: ₹120 per night
1️⃣8️⃣ Flight Ticket Pricing
✈️ Write a program that takes the flight distance and class of service as input and outputs the ticket price based on the following conditions:
- first class and distance above 1000 miles: ₹1000
- business class and distance above 1000 miles: ₹800
- economy class and distance above 1000 miles: ₹500
- first class and distance below 1000 miles: ₹800
- business class and distance below 1000 miles: ₹600
- economy class and distance below 1000 miles: ₹400
1️⃣9️⃣ Car Insurance Quote
🚗 Write a program that takes the driver’s age and vehicle value as input and outputs the insurance quote based on the following conditions:
- driver above 25 and vehicle value above ₹20000: ₹100 per month
- driver above 25 and vehicle value below ₹20000: ₹80 per month
- driver below 25 and vehicle value above ₹20000: ₹150 per month
- driver below 25 and vehicle value below ₹20000: ₹120 per month
2️⃣0️⃣ Student Loan Interest Rate
💸 Write a program that takes the student’s GPA and loan amount as input and outputs the interest rate based on the following conditions:
- GPA above 3.5 and loan amount above ₹10000: 4%
- GPA above 3.0 and loan amount above ₹5000: 5%
- GPA above 2.5 and loan amount above ₹2000: 6%
- otherwise: 7%
2️⃣1️⃣ Taxi Fare Calculator
🚖 Write a program that takes the distance traveled and time of day as input and outputs the taxi fare based on the following conditions:
- distance above 10 miles and peak hours (7 - 9 am, 4 - 7 pm): ₹25 + ₹2 per mile
- distance above 10 miles and off-peak hours: ₹20 + ₹1.50 per mile
- distance below 10 miles and peak hours: ₹15 + ₹2 per mile
- distance below 10 miles and off-peak hours: ₹10 + ₹1.50 per mile
2️⃣2️⃣ Bank Transaction Fees
🏧 Write a program that takes the transaction type and amount as input and outputs the fee based on the following conditions:
- withdrawal and amount above ₹1000: ₹5
- withdrawal and amount below ₹1000: ₹2
- deposit and amount above ₹1000: ₹0
- deposit and amount below ₹1000: ₹1
2️⃣3️⃣ Mobile Phone Plan
📱 Write a program that takes the data usage and number of minutes as input and outputs the monthly bill based on the following conditions:
- data above 10GB and minutes above 1000: ₹100
- data above 10GB and minutes below 1000: ₹80
- data below 10GB and minutes above 1000: ₹70
- data below 10GB and minutes below 1000: ₹50
2️⃣4️⃣ Restaurant Bill Calculator
🍽️ Write a program that takes the total bill and number of guests as input and outputs the bill per person based on the following conditions:
- bill above ₹100 and more than 5 guests: split equally
- bill above ₹100 and 2 - 5 guests: add 15% gratuity
- bill below ₹100 and more than 5 guests: split equally
- bill below ₹100 and 2 - 5 guests: add 10% gratuity
2️⃣5️⃣ Travel Insurance Quote
🌍 Write a program that takes the trip cost and age of traveler as input and outputs the insurance quote based on the following conditions:
- trip cost above ₹5000 and age above 60: ₹200
- trip cost above ₹5000 and age below 60: ₹150
- trip cost below ₹5000 and age above 60: ₹100
- trip cost below ₹5000 and age below 60: ₹50
2️⃣6️⃣ Electricity Bill Calculator
⚡ Write a program that takes the units consumed and type of connection as input and outputs the electricity bill based on the following conditions:
- domestic connection and units above 500: ₹1.50 per unit
- domestic connection and units below 500: ₹1.20 per unit
- commercial connection and units above 1000: ₹2.00 per unit
- commercial connection and units below 1000: ₹1.80 per unit
2️⃣7️⃣ Water Bill Calculator
💧 Write a program that takes the gallons consumed and type of user as input and outputs the water bill based on the following conditions:
- residential user and gallons above 10000: ₹5.00 per 1000 gallons
- residential user and gallons below 10000: ₹4.00 per 1000 gallons
- commercial user and gallons above 50000: ₹10.00 per 1000 gallons
- commercial user and gallons below 50000: ₹8.00 per 1000 gallons
2️⃣8️⃣ Internet Plan Selector
🌐 Write a program that takes the data usage and speed required as input and outputs the recommended internet plan based on the following conditions:
- data above 100GB and speed above 100 Mbps: Premium Plan
- data above 50GB and speed above 50 Mbps: Standard Plan
- data below 50GB and speed below 50 Mbps: Basic Plan
2️⃣9️⃣ Loan Repayment Calculator
💳 Write a program that takes the loan amount and repayment period as input and outputs the monthly repayment amount based on the following conditions:
- loan above ₹10000 and repayment period above 5 years: ₹200 per month
- loan above ₹5000 and repayment period above 3 years: ₹150 per month
- loan below ₹5000 and repayment period below 3 years: ₹100 per month
3️⃣0️⃣ Health Insurance Premium Calculator
🏥 Write a program that takes the age and medical history as input and outputs the health insurance premium based on the following conditions:
- age above 60 and medical history: ₹500 per month
- age above 50 and medical history: ₹300 per month
- age below 50 and no medical history: ₹200 per month
3️⃣1️⃣ Credit Score Calculator
📈 Write a program that takes the credit history and income as input and outputs the credit score based on the following conditions:
- credit history above 7 years and income above ₹50000: 750
- credit history above 5 years and income above ₹30000: 700
- credit history below 5 years and income below ₹30000: 650
3️⃣2️⃣ Tuition Fee Calculator
🎓 Write a program that takes the program type and residency status as input and outputs the tuition fee based on the following conditions:
- international student and graduate program: ₹20000
- domestic student and graduate program: ₹15000
- international student and undergraduate program: ₹18000
- domestic student and undergraduate program: ₹12000
3️⃣3️⃣ Sales Commission Calculator
💼 Write a program that takes the sales amount and region as input and outputs the sales commission based on the following conditions:
- sales above ₹10000 and region A: 10%
- sales above ₹5000 and region B: 8%
- sales below ₹5000 and region C: 5%
3️⃣4️⃣ Hotel Room Booking System
🏨 Write a program that takes the room type and number of guests as input and outputs the room rent based on the following conditions:
- 🛌 room type deluxe and number of guests above 2: ₹5000
- 🛌 room type deluxe and number of guests below 2: ₹3000
- 🏨 room type standard and number of guests above 2: ₹3000
- 🏨 room type standard and number of guests below 2: ₹2000
3️⃣5️⃣ Flight Seat Selection
✈️ Write a program that takes the seat preference and class of service as input and outputs the seat selection based on the following conditions:
- seat preference window and class of service economy: 10A
- seat preference aisle and class of service economy: 10B
- seat preference window and class of service business: 1A
- seat preference aisle and class of service business: 1B
3️⃣6️⃣ Car Rental Selection
🚗 Write a program that takes the rental duration and car type as input and outputs the rental selection based on the following conditions:
- rental duration above 7 days and car type sedan: Toyota Camry
- rental duration above 7 days and car type SUV: Honda CRV
- rental duration below 7 days and car type sedan: Honda Civic
- rental duration below 7 days and car type SUV: Toyota RAV4
3️⃣7️⃣ Health Club Membership Fee Calculator
🏋️ Write a program that takes the membership type and age as input and outputs the membership fee based on the following conditions:
- membership type premium and age above 60: ₹100
- membership type premium and age below 60: ₹80
- membership type basic and age above 60: ₹60
- membership type basic and age below 60: ₹40
3️⃣8️⃣ Phone Plan Selection
📞 Write a program that takes the data usage and call minutes as input and outputs the phone plan selection based on the following conditions:
- data usage above 10GB and call minutes above 1000: Unlimited Plan
- data usage above 5GB and call minutes above 500: Standard Plan
- data usage below 5GB and call minutes below 500: Basic Plan
3️⃣9️⃣ Travel Agency Commission Calculator
🌍 Write a program that takes the travel package and agency type as input and outputs the agency commission based on the following conditions:
- travel package luxury and agency type online: 10%
- travel package standard and agency type online: 8%
- travel package luxury and agency type offline: 12%
- travel package standard and agency type offline: 10%
4️⃣0️⃣ Insurance Policy Premium Calculator
🛡️ Write a program that takes the policy type and age as input and outputs the policy premium based on the following conditions:
- policy type life insurance and age above 60: ₹500
- policy type life insurance and age below 60: ₹300
- policy type health insurance and age above 60: ₹400
- policy type health insurance and age below 60: ₹200
Hope you find this helpful. Happy coding!
Will keep updating these list.